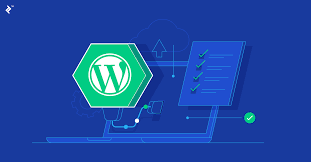
For expert developers working with WordPress and PHP, following best practices is crucial for building secure, scalable, and maintainable code. Here are some recommended best practices:
Use Child Themes
When customizing a theme, create a child theme instead of modifying the original theme files. This ensures that your changes are not lost when the theme is updated.
// Example child theme style.css
/*
Theme Name: Twenty Twenty Child
Template: twentytwenty
*/
Use Hooks and Filters
Leverage the power of hooks and filters to modify and extend WordPress functionality. This allows you to customize themes and plugins without directly editing their code.
Secure Your Code
Sanitize and validate user input to prevent security vulnerabilities like SQL injection and Cross-Site Scripting (XSS) attacks. Use functions like esc_html
, esc_attr
, sanitize_text_field
, and others to sanitize user input.
$input = sanitize_text_field($_POST['user_input']);
Use Custom Post Types and Taxonomies
When creating content types beyond posts and pages, use custom post types and taxonomies. This enhances organization and allows for better control over the content structure.
Optimize Database Queries
Optimize database queries for better performance. Use the $wpdb
class for custom queries and make use of indexes where necessary. Avoid using SELECT *
and fetch only the required fields.
$results = $wpdb->get_results("SELECT column1, column2 FROM $wpdb->custom_table WHERE condition = 'value'");
Implement Caching
Implement caching mechanisms to reduce server load and improve site speed. Use caching plugins or implement object caching with tools like Memcached or Redis.
Version Control
Use version control systems like Git to track changes in your code. This helps in collaboration, rollback, and code maintenance.
Organize Code Structure
Maintain a clean and organized code structure. Group related functions together, use proper indentation, and add comments for clarity.
Performance Optimization
Optimize your code for performance by minimizing the number of HTTP requests, leveraging browser caching, and optimizing images. Use tools like PageSpeed Insights for performance analysis.
Update Regularly
Keep WordPress, themes, and plugins up to date to benefit from the latest features, security patches, and improvements.
Use Composer for Dependency Management
If your project involves external libraries or packages, use Composer for dependency management. This helps manage and autoload dependencies efficiently.
Error Handling
Implement robust error handling to gracefully handle errors and log them for debugging purposes. Use try-catch
blocks for critical sections of your code.
try {
// Code that might throw an exception
} catch (Exception $e) {
// Handle the exception
error_log('An error occurred: ' . $e->getMessage());
}
By adhering to these best practices, you can build maintainable and secure WordPress applications while optimizing performance and ensuring scalability.